In this post we’ll show you how to configure network adapter parameters in Windows via PowerShell. We will learn how to get and set a static IP address and DNS (nameservers) and configure your network interface to obtain the IP configuration from a DHCP server. You can use these cmdlets to configure network in Core/Nano versions of Windows Server, in Hyper-V Server, to change IP settings on remote computers/servers and in your PS scripts.
Previously, the netsh interface ipv4
command was used to configure Windows network settings from the CLI. In PowerShell 3.0 and newer, you can use the built-in PowerShell NetTCPIP module to manage Windows network settings.
To get the list of cmdlets in this module, run the following command:
get-command -module NetTCPIP
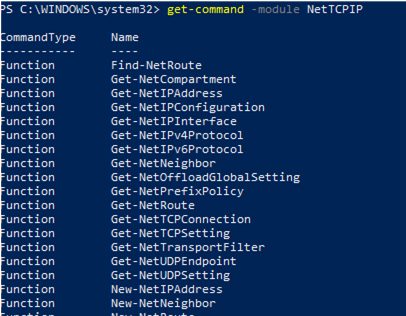
This module also includes the Test-NetConnection cmdlet you can use to test TCP port availability on remote computers.
Managing Network Adapters with PowerShell
Display the list of available network interfaces on a computer:
Get-NetAdapter
The cmdlet returns the interface name, its state (Up/Down), MAC address and port speed.
In this example, I have multiple network adapters on my computer (besides the physical connection, Ethernet0, I have some Hyper-V and VMWare Player network interfaces).

You can refer network interfaces by their names or indexes (the Index column). In our example, to select the physical LAN adapter Intel 82574L, use the command:
Get-NetAdapter -Name "Ethernet0"
or:
Get-NetAdapter -InterfaceIndex 8

You can change the adapter name:
Rename-NetAdapter -Name Ethernet0 -NewName LAN
To disable a network interface, use this command:
Get-NetAdapter -InterfaceIndex 13| Disable-NetAdapter
When you enable an interface, you cannot use its index since it is not assigned yet. You can specify an adapter name or description:
Enable-NetAdapter -InterfaceDescription “Hyper-V Virtual Ethernet Adapter"
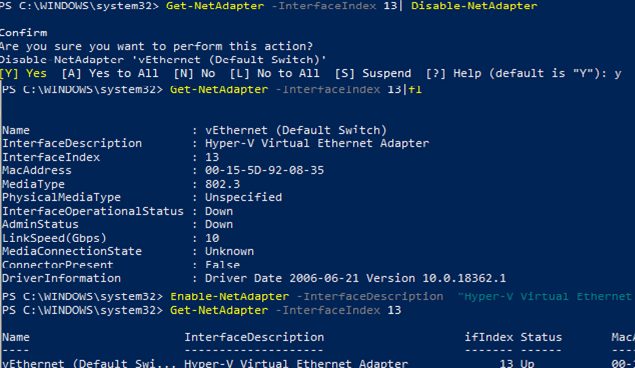
If a VLAN is specified for an adapter, you can display it as follows:
Get-NetAdapter | ft Name, Status, Linkspeed, VlanID
Here is how you can get the information about the network adapter driver used:
Get-NetAdapter | ft Name, DriverName, DriverVersion, DriverInformation, DriverFileName

The information about physical network adapters (PCI slot, bus, etc.):
Get-NetAdapterHardwareInfo
How to View TCP/IP Network Adapter Settings with PowerShell?
To get current network adapter settings (IP address, DNS, default gateway):
Get-NetIPConfiguration -InterfaceAlias Ethernet0
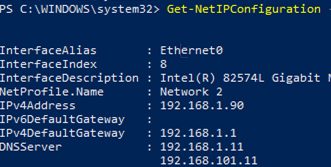
To display a detailed information about current network adapter TCP/IP configuration, use this command:
Get-NetIPConfiguration -InterfaceAlias Ethernet0 -Detailed
In this case, the assigned network profile (NetProfile.NetworkCategory) of the interface, MTU settings (NetIPv4Interface.NlMTU), whether obtaining an IP address from DHCP is enabled (NetIPv4Interface.DHCP) and other useful information are displayed.
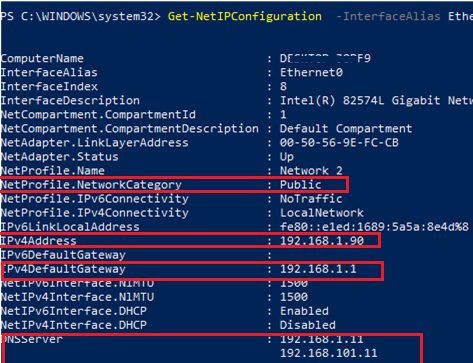
To get the interface IPv4 address only:
(Get-NetAdapter -Name ethernet0 | Get-NetIPAddress).IPv4Address
Using PowerShell to Set Static IP Address
Let’s try to set a static IP address for the NIC. To change an IP address, a subnet mask and default gateway for a network interface use:
New-NetIPAddress –IPAddress 192.168.2.50 -DefaultGateway 192.168.2.1 -PrefixLength 24 -InterfaceIndex 8
You can set an IP address using an array structure (more visually):
$ipParams = @{
You can use the New-NetIPAddress to add a second IP address (alias) to a network adapter.
InterfaceIndex = 8
IPAddress = "192.168.2.50"
PrefixLength = 24
AddressFamily = "IPv4"
}
New-NetIPAddress @ipParams
If the static IP address has already been configured and you want to change it, the Set-NetIPAddress cmdlet is used:
Set-NetIPAddress -InterfaceIndex 8 -IPAddress 192.168.2.90
To disable obtaining an IP address from DHCP for your adapter, run the command:
Set-NetIPInterface -InterfaceAlias Ethernet0 -Dhcp Disabled
To view the routing table, the Get-NetRoute cmdlet is used. To add a new route, use the New-NetRoute cmdlet:
New-NetRoute -DestinationPrefix "0.0.0.0/0" -NextHop "192.168.2.2" -InterfaceIndex 8
To disable the IPv6 protocol for the network adapter:
Get-NetAdapterBinding -InterfaceAlias Ethernet0 | Set-NetAdapterBinding -Enabled:$false -ComponentID ms_tcpip6
Set-DnsClientServerAddress: Set Primary and Secondary DNS Server Addresses
In order to set the primary and secondary DNS server IP addresses in Windows, use the Set-DNSClientServerAddress cmdlet. For example:
Set-DNSClientServerAddress –InterfaceIndex 8 –ServerAddresses 192.168.2.11,10.1.2.11
You can also set nameservers using an array:
$dnsParams = @{
InterfaceIndex = 8
ServerAddresses = ("8.8.8.8","8.8.4.4")
}
Set-DnsClientServerAddress @dnsParams
After changing your DNS settings, you can clear the resolver cache:
Clear-DnsClientCache
How to Change Static IP Address to DHCP using PowerShell?
To allow the computer to obtain a dynamic IP address from the DHCP server for the network adapter, run this command:
Set-NetIPInterface -InterfaceAlias Ethernet0 -Dhcp Enabled
Clear the DNS server settings:
Set-DnsClientServerAddress –InterfaceIndex 8 -ResetServerAddresses
And restart your adapter in order to obtain an IP address automatically from the DHCP server:
Restart-NetAdapter -InterfaceAlias Ethernet0
If you previously had a default gateway configured, remove it:
Set-NetIPInterface -InterfaceAlias Ethernet0| Remove-NetRoute -Confirm:$false
How to Remotely Change IP Address and DNS settings with PowerShell?
You can use PowerShell to remotely change IP address or DNS server settings on multiple remote computers. Suppose, your task is to change DNS settings for all servers in the specific AD container (Organizational Unit). To get the list of computers in the script below, the Get-ADComputer cmdlet is used, and WinRM is used to connect to computers remotely (the Invoke-Command cmdlet):
$Servers = Get-ADComputer -SearchBase ‘OU=Servers,DC=contoso,DC=com’ -Filter '(OperatingSystem -like "Windows Server*")' | Sort-Object Name
ForEach ($Server in $Servers) {
Write-Host "Server $($Server.Name)"
Invoke-Command -ComputerName $Server.Name -ScriptBlock {
$NewDnsServerSearchOrder = "192.168.2.11","8.8.8.8"
$Adapters = Get-WmiObject Win32_NetworkAdapterConfiguration | Where-Object {$_.DHCPEnabled -ne 'True' -and $_.DNSServerSearchOrder -ne $null}
Write-Host "Old DNS settings: "
$Adapters | ForEach-Object {$_.DNSServerSearchOrder}
$Adapters | ForEach-Object {$_.SetDNSServerSearchOrder($NewDnsServerSearchOrder)} | Out-Null
$Adapters = Get-WmiObject Win32_NetworkAdapterConfiguration | Where-Object {$_.DHCPEnabled -ne 'True' -and $_.DNSServerSearchOrder -ne $null}
Write-Host "New DNS settings: "
$Adapters | ForEach-Object {$_.DNSServerSearchOrder}
}
}